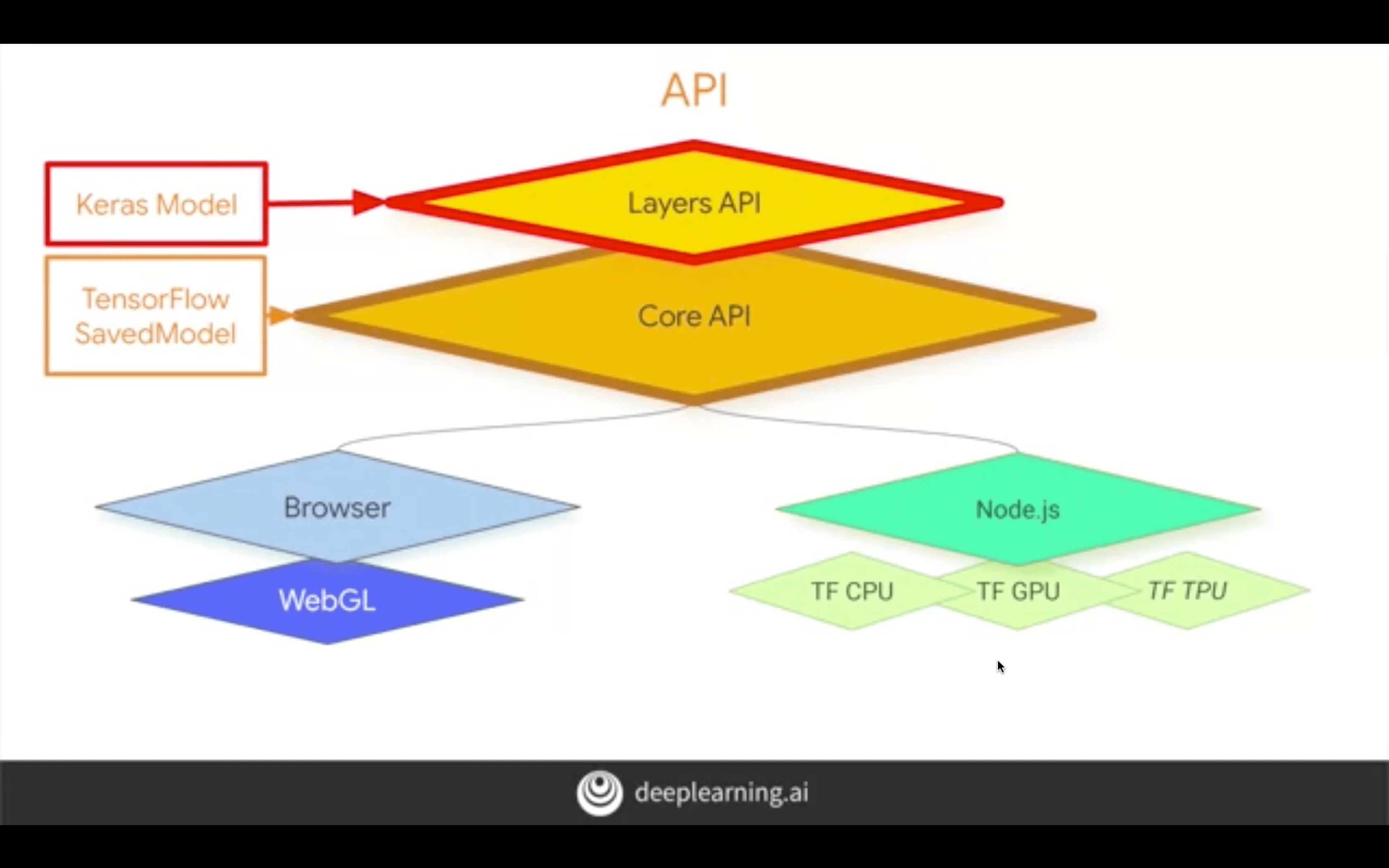
GitHub 源码:https://github.com/GameDevLog/TensorFlow-Data-and-Deployment-Specialization
1. 使用TensorFlow.js的基于浏览器的模型(Browser-based Models with TensorFlow.js)
将机器学习模型带入现实世界不仅仅涉及建模。本专业知识将教您如何导航各种部署方案并更有效地使用数据来训练模型。在第一门课程中,您将使用TensorFlow.js在任何浏览器中训练和运行机器学习模型。您将学习在浏览器中处理数据的技术,最后将建立一个计算机视觉项目,该项目可以识别和分类来自网络摄像头的对象。该专业化基于我们的TensorFlow实践专业化。如果您不熟悉TensorFlow,我们建议您首先参加TensorFlow实践专业化课程。为了深入了解神经网络的工作原理,我们建议您参加“深度学习专业化”课程。
Building the Model
First HTML Page
1 | <html> |
tfjs script
1 |
|
model
1 |
|
data
1 |
|
train
1 |
|
doTraining
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24 @@ -4,6 +4,23 @@
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@latest"></script>
<script lang="js">
+ async function doTraining(model) {
+ const history =
+ await model.fit(xs, ys,
+ {
+ epochs: 500,
+ callbacks: {
+ onEpochEnd: async (epoch, logs) => {
+ console.log("Epoch:"
+ + epoch
+ + " Loss:"
+ + logs.loss);
+
+ }
+ }
+ });
+ }
+
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [1] }));
model.compile({
test
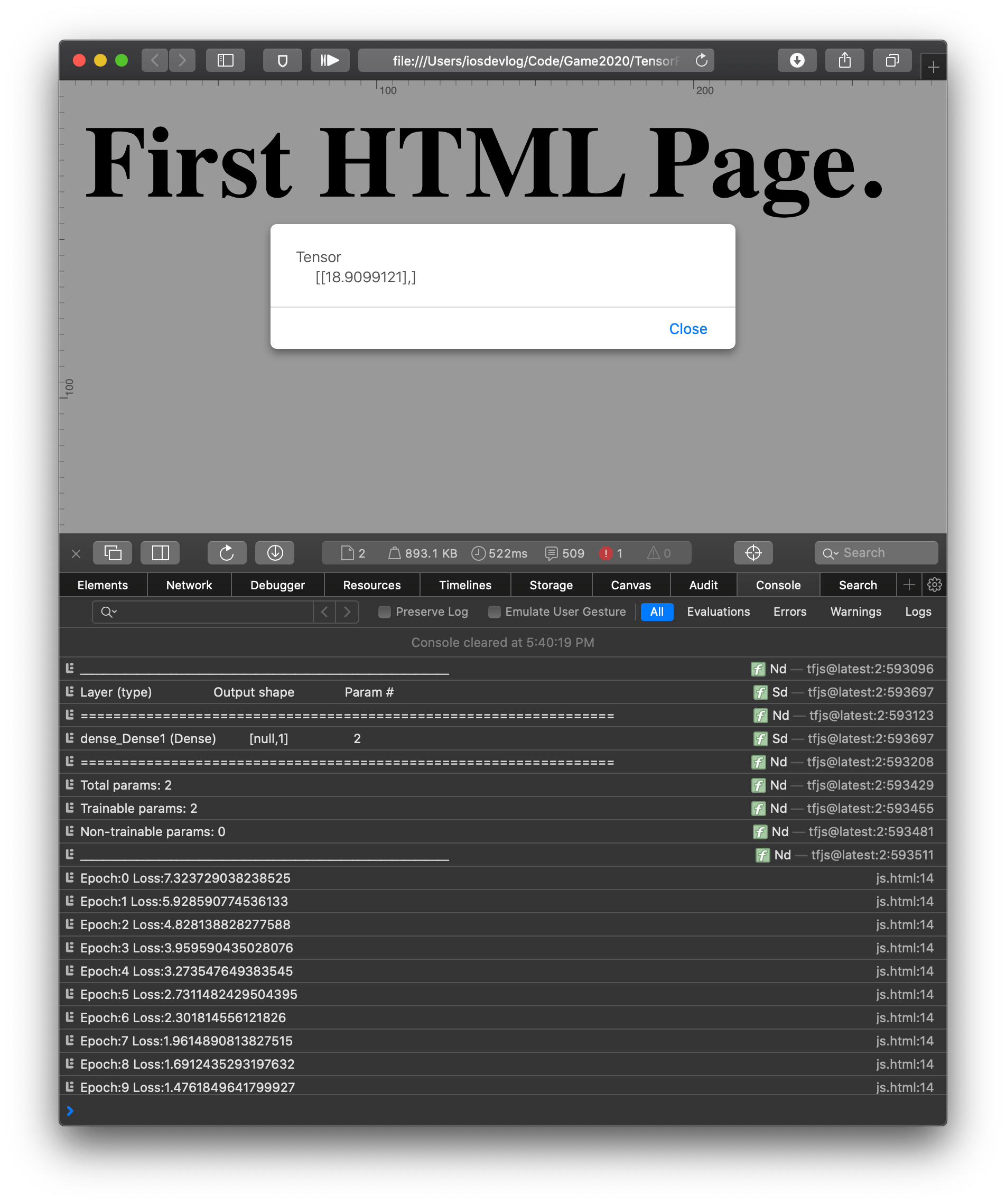
Iris
https://archive.ics.uci.edu/ml/datasets/Iris
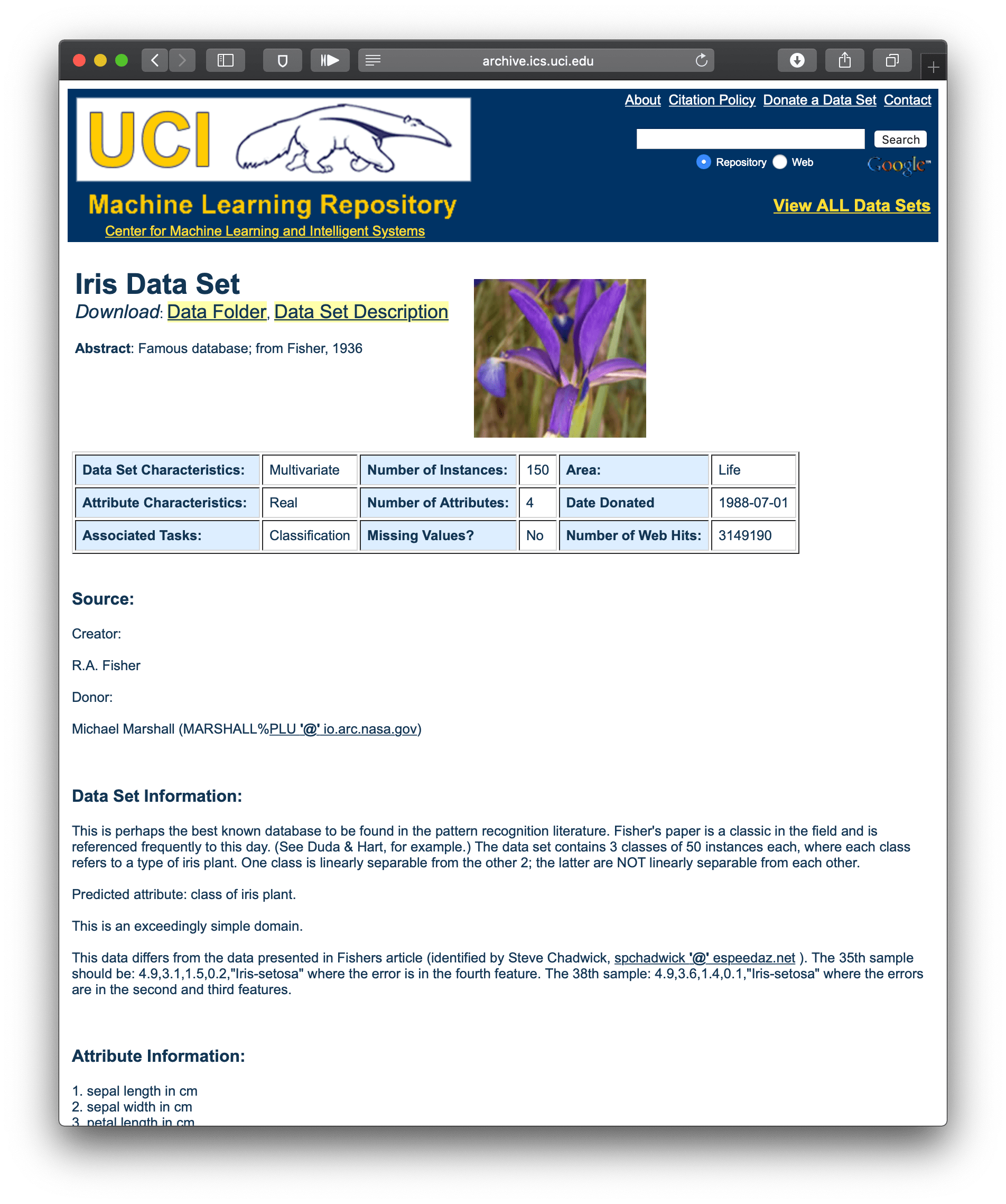
https://commons.wikimedia.org/w/index.php?curid=46257808
iris.csv
1 | sepal_length,sepal_width,petal_length,petal_width,species |
async
1 |
|
### load iris.csv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17 @@ -3,6 +3,16 @@
<head></head>
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@latest"></script>
<script lang="js">
+ async function run() {
+ const csvUrl = 'iris.csv';
+ const trainingData = tf.data.csv(csvUrl, {
+ columnConfigs: {
+ species: {
+ isLabel: true
+ }
+ }
+ });
+ }
</script>
<body>
One-Hot encoder
1 |
|
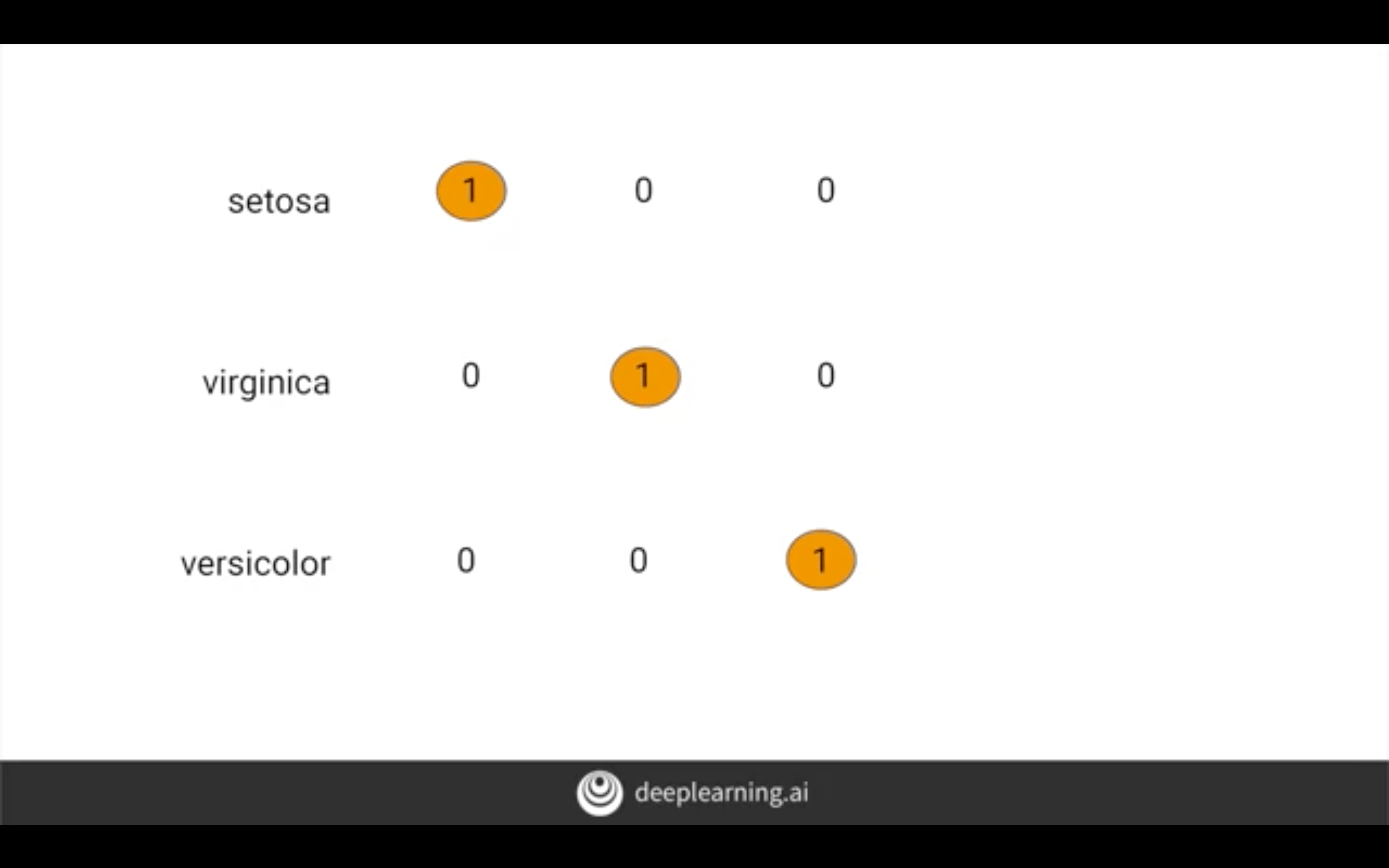
NN
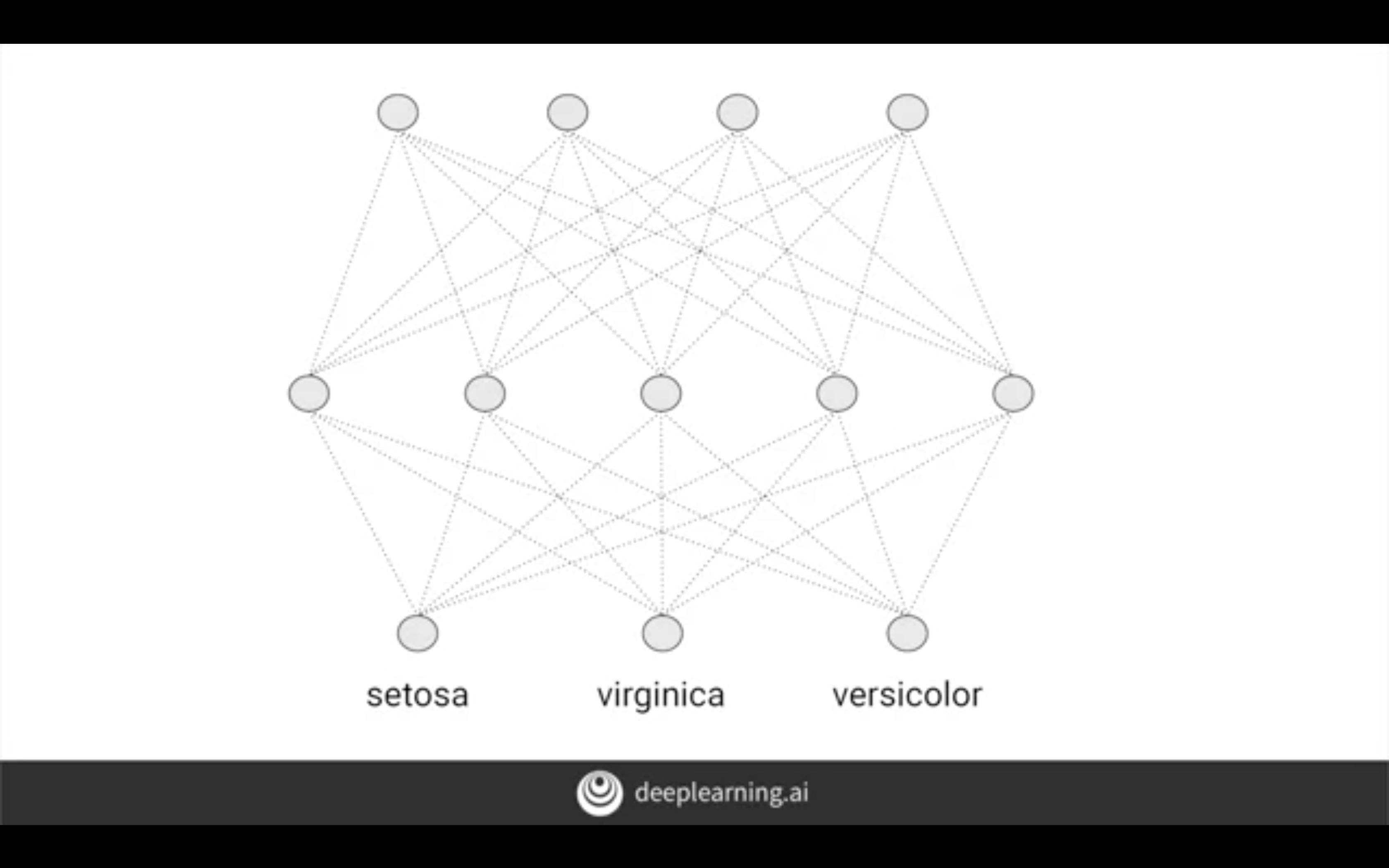
1 |
|
compile
1 |
|
fit
1 |
|
### predict
1
2
3
4
5
6
7
8
9
10
}
}
});
+
+ const testVal = tf.tensor2d([5.8, 2.7, 5.1, 1.9], [1, 4]);
+ const prediction = model.predict(testVal);
+ alert(prediction)
}
</script>
run
1 |
|
HTTP Server
1
python2 -m SimpleHTTPServer
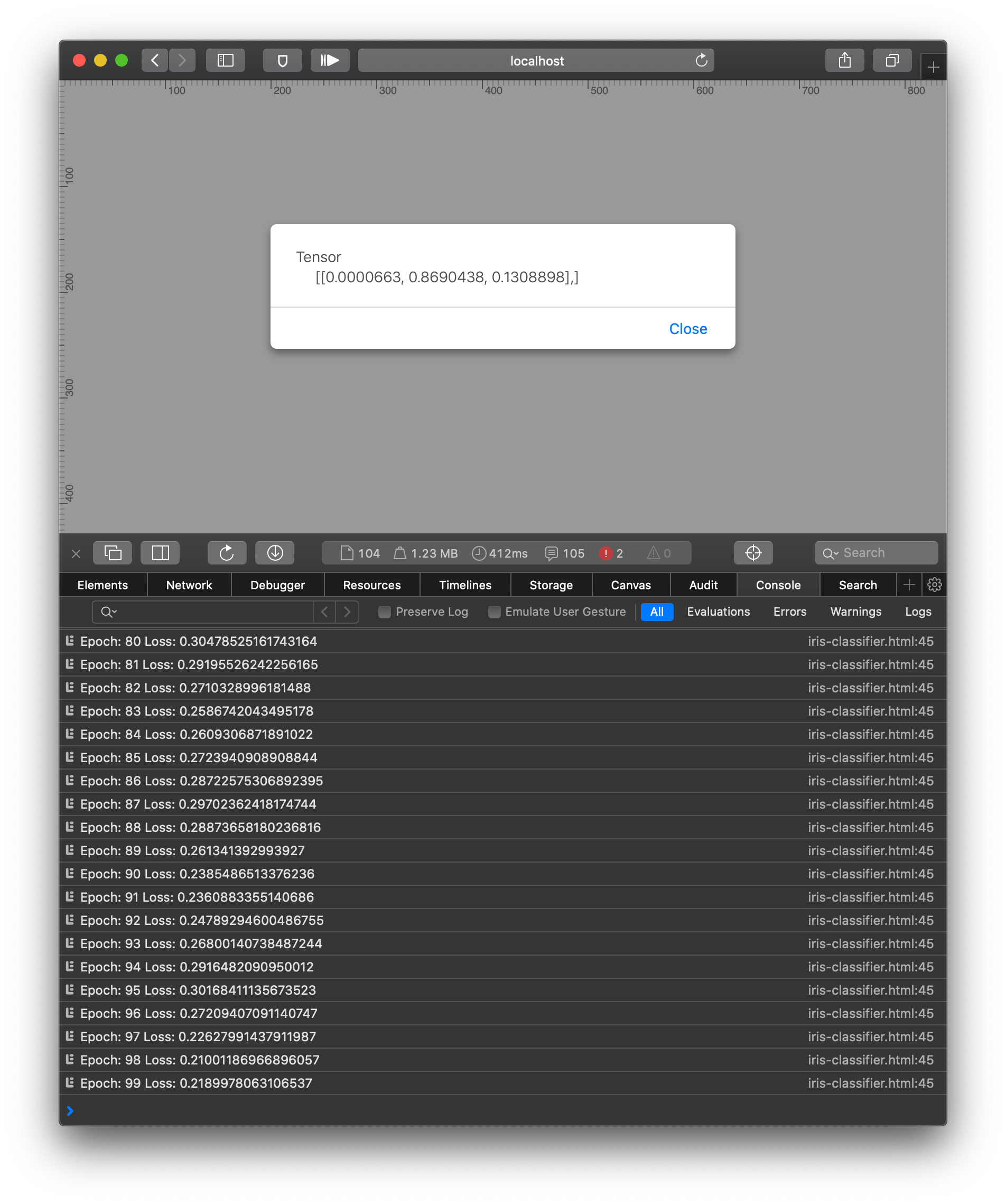
第 2 种概率最高
1 | Tensor |
className
1 |
|
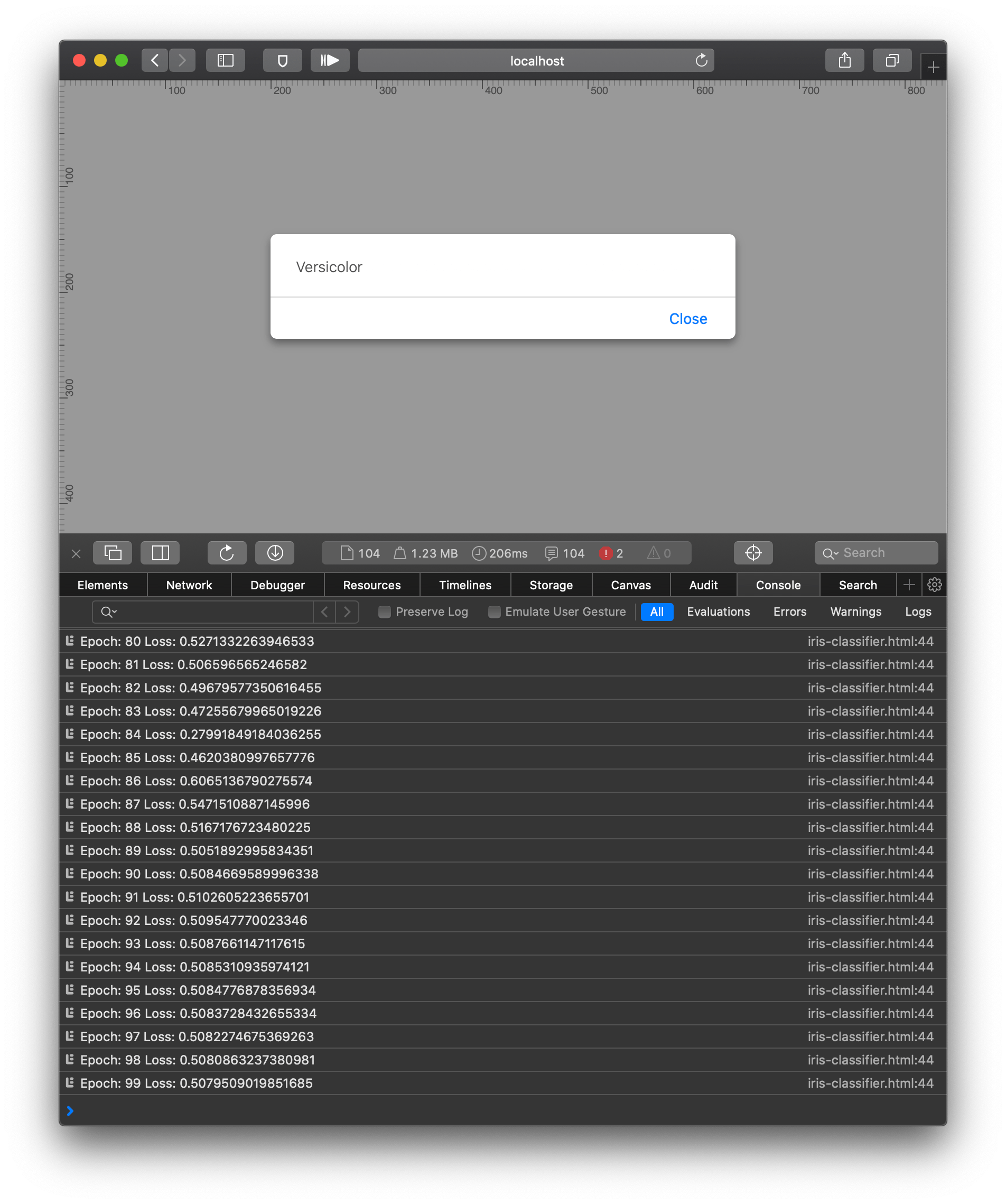
2. 使用TensorFlow Lite的基于设备的模型(Device-based Models with TensorFlow Lite)
将机器学习模型带入现实世界不仅仅涉及建模。本专业知识将教您如何导航各种部署方案并更有效地使用数据来训练模型。第二门课程教您如何在移动应用程序中运行机器学习模型。您将学习如何为低功耗,电池供电的设备准备模型,然后在Android和iOS平台上执行模型。最后,您将探索如何在Raspberry Pi和微控制器上使用TensorFlow在嵌入式系统上进行部署。该专业化基于我们的TensorFlow实践专业化。如果您不熟悉TensorFlow,我们建议您首先参加TensorFlow实践专业化课程。为了深入了解神经网络的工作原理,
3. 使用TensorFlow数据服务的数据管道(Data Pipelines with TensorFlow Data Services)
将机器学习模型带入现实世界不仅仅涉及建模。本专业知识将教您如何导航各种部署方案并更有效地使用数据来训练模型。在这第三门课程中,您将在TensorFlow中使用一套工具来更有效地利用数据和训练模型。您将学习如何仅用几行代码就可以利用内置数据集,如何使用API控制如何拆分数据以及如何处理所有类型的非结构化数据。该专业化基于我们的TensorFlow实践专业化。如果您不熟悉TensorFlow,我们建议您首先参加TensorFlow实践专业化课程。为了深入了解神经网络的工作原理,我们建议您参加“深度学习专业化”课程。
4. 使用TensorFlow的高级部署方案(Advanced Deployment Scenarios with TensorFlow)
将机器学习模型带入现实世界不仅仅涉及建模。本专业知识将教您如何导航各种部署方案并更有效地使用数据来训练模型。在这最后的课程中,您将探索在部署模型时会遇到的四种不同情况。将向您介绍TensorFlow Serving,该技术可让您通过Web进行推理。您将继续使用TensorFlow Hub,该模型库可用于转移学习。然后,您将使用TensorBoard评估并了解模型的工作方式,并与他人共享模型元数据。最后,您将探索联合学习,以及如何在保持数据隐私的同时使用用户数据重新训练已部署的模型。该专业化基于我们的TensorFlow实践专业化。如果您不熟悉TensorFlow,我们建议您首先参加TensorFlow实践专业化课程。为了深入了解神经网络的工作原理,我们建议您参加“深度学习专业化”课程。